Java REST (Representational State Transfer) is a most common way to expose web services, but how can any developer or third party will understand or utilize those services easily? There is no such straightforward rules or standards to write those services.
To use web services by third person, we have to manually create document and it will be very difficult to keep perfectly synchronized that document with the API.
Key points to maintain API documentation.
- URL's.
- Input format - JSON
- Input data types - String, Integer, Date .... etc
- Output.
- Error codes and messages.
Developer can write their web services using any popular framework, and top of the web services they needs to define some simple annotation provided by swagger, when application will start swagger scans your code and exposes documentation based on the defined annotation. Without an extra efforts any developer, tester or client can consume this URL and learn how to use web services or can test this web services.
To use web services by third person, we have to manually create document and it will be very difficult to keep perfectly synchronized that document with the API.
Key points to maintain API documentation.
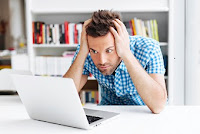
- Input format - JSON
- Input data types - String, Integer, Date .... etc
- Output.
- Error codes and messages.
To overcome all these challenges, there is another way to create documentation in the code it self by using Swagger framework.
To overcome all these challenges, there is another way to create documentation in the code it self by using Swagger framework.
Swagger
Swagger is a simple yet powerful representation of your RESTful API, Swagger defines standard language interface to REST APIs which allow both humans and computers to discover and understand the capabilities of the service without access to source code, documentation.Developer can write their web services using any popular framework, and top of the web services they needs to define some simple annotation provided by swagger, when application will start swagger scans your code and exposes documentation based on the defined annotation. Without an extra efforts any developer, tester or client can consume this URL and learn how to use web services or can test this web services.
Implementation of Swagger in Java REST services
Consider below simple Rest service which accepts input as a String and return back response in String.
@GET
@Path("/printMessage")
@Produces(MediaType.APPLICATION_JSON)
public String printMessage(String msg) {
return "Hello : " + msg;
}
To export this service to third party, you need to provide documentation saying the URL, Request type, Input parameters, Response type, Data types .... etc
Now by using swagger we can easily show all above information, please look below image stating all information about above Rest service.
Swagger URL for this application - http://localhost:8080/RestEasy
By looking at above image you have all the information about above Rest service, no need to create manually documentation.
- Name of the Rest service with description.
- URL with description.
- Type of Rest service - GET.
- Return response - String class
- Number of parameters with description and data type.
- All the list of operations.
Now to implement Swagger we need to follow below simple steps.
Code is already checked-in to Git repository - Developer-Tech
- Add pom dependency.
<dependency>
<groupId>com.wordnik</groupId>
<artifactId>swagger-jaxrs_2.9.1</artifactId>
<version>1.3.0</version>
</dependency>
- Show Swagger ui page - Please copy css, images, lib, index.html, swagger-ui.js, swagger-ui.min.js files in to webapp folder.
- SwaggerRestServiceApplication.java - This is main file is used to create singletons object of Rest service class.
- web.xml - Depends on your service call please make necessary changes in your web.xml.
<servlet>
<servlet-name>DefaultJaxrsConfig</servlet-name>
<servlet-class>com.wordnik.swagger.jaxrs.config.DefaultJaxrsConfig</servlet-class>
<init-param>
<param-name>api.version</param-name>
<param-value>1.0.0</param-value>
</init-param>
<init-param>
<param-name>swagger.api.basepath</param-name>
<param-value>http://localhost:8080/RestEasy</param-value>
</init-param>
<load-on-startup>2</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>resteasy-servlet</servlet-name>
<url-pattern>/swagger/*</url-pattern>
<url-pattern>/api-docs/*</url-pattern>
</servlet-mapping>
Finally please add Swagger annotation
- SwaggerRestService.java - For more information regarding annotation please refer Swagger Annotations.
Code is available in Git repository - Developer-Tech
For more information, please feel free to contact me.